Virtual Device API
A Thing-App shall be able to interact with end users during runtime.
Libertas OS introduces virtual devices for interaction between Thing-Apps and end-users. Virtual devices give users extra control over the Thing-App during runtime.
Libertas OS defines a set of APIs to drive the virtual devices.
Virtual device API is reactive only.
Shared Life with Thing-App Tasks
Virtual devices are associated with corresponding Thing-App tasks.
When an end-user creates a Thing-App task, a set of new virtual devices may be created along with the task. End-users then use those virtual devices to interact with the Thing-App task during runtime.
The associated virtual devices are also automatically removed when the Thing-App task is removed.
Hub Virtual Device and Node Virtual Device
If the task is running on Hub, the corresponding virtual devices will be driven by Hub.
If the task is running on a device node, some extra endpoints will be dynamically created on the node and the virtual devices will be driven by the node.
The network will be automatically configured with updated node data, routing and binding information. Libertas invented interaction and interconnection, virtual device is part of our inventions.
Creating Virtual Devices
Developer
Developer’s Code
A developer can define many virtual devices as part of an entry function’s input arguments (inside a list of tree structures). When an end-user creates a Thing-App task based on the function, the developer-defined virtual devices are automatically created as new devices.
export enum DummyStates {
State1,
State2,
State3,
}
export function VirtualDevicesDemo(
dummyOnOff: LibertasVirtualDevice,
dummyLevel: LibertasVirtualDevice,
dummyMultiStates: LibertasVirtualDevice,
dummyOnOffList: LibertasVirtualDevice[],
dummyOptionalOnOff?: LibertasVirtualDevice) {
The code defined virtual devices as input arguments.
- All devices are dummy devices, meaning that it will acknowledge any request without actually doing anything.
- The first three devices are on/off, level control, and multi-state.
- The fourth argument is an array (List) of on/off devices. End-users can create many virtual devices.
- The fifth argument is an optional on/off device. The end-user chooses whether or not to create this virtual device.
Developer Specifying Virtual Devie Types
All devices are of LibertasVirtualDevice
type. The developer shall further specify the type of each virtual device using the Signature Editor tool.
Signature Editor will pop up when a developer chooses to “Deploy” the Thing-App code.
Developer’s Enum and Multi-state Device
An on/off device only has two states, on and off. A multi-state device can have more than two states.
Libertas Signature Editor requires a developer to associate an enum declaration with a multi-state device. In this example, we associate DummyEnums
with dummyMultiStates
device.
The text of each enumeration can be customized or translated into other languages using Libertas Signature Editor. Text customization will be reflected on the end-user UI.
End-user
End-user Configuration
An end-user can create a Thing-App task of the VirtualDevicesDemo
function. At first, it may seem counter-intuitive that virtual devices are part of end-user input data. Nevertheless, please remember that virtual devices are created with Thing-App tasks. The user who created Thing-App tasks also created virtual devices associated with the task.
During configuration, end-users may change each virtual device’s name and icon. End-users can also create extra virtual devices inside a list (array) or originally as optional.
End-user Runtime
During runtime, end-users interact with Thing-App using virtual devices.
The animation below demonstrates an end-user using the virtual devices in the above example. The end-user changed the level of the “MyDummyLevel” to 20%, then changed the state of “MyDummyMultiStates” into “State2”.
Click on the animation below to play.
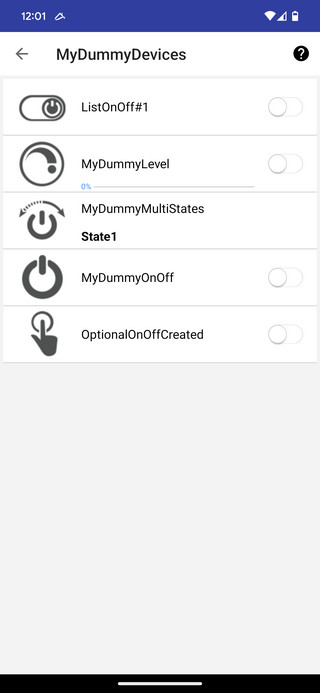
Evolving Over Time
Virtual device is a way to enable any possible runtime interaction between users and Thing-Apps.
Presentation is relatively cheap. And presentation will be improved over time.
More device types will be added to the standard, making more versatile virtual devices.
Virtual Device as Device Driver
A Thing-App function may work as a driver that turns other TCP/IP controllable devices into Libertas-compatible devices.
The diagram below demonstrates a driver for Denon/Marantz receivers. A user creates a task and throws a receiver on the local network. The task will expose a list of virtual devices, including:
- An “On/Off” power device
- A “Level Control” volume devices
- Several “Multi-state” devices for input/output channel selection, Surround mode selection, etc
- If multi-zone is used, devices for extra zones will also be exposed
Once the user creates a task to drive a Denon receiver, several new devices will be created in the system. The user can even specify the device name and icon during the configuration time, as shown on the left of the diagram.
The middle of the diagram is a screenshot of virtual devices on the device control screen during the task’s runtime. For example, a user can control the virtual devices by changing the volume.
Virtual Devices as First-Class Citizens
Virtual devices are treated the same as any other type of device. Although “virtual,” it can only be initially controlled from smartphone UI.
However, later on, an end user can “bind” a virtual with a real device to make a virtual device physical. For example, an end user may create a binding between a “virtual button” and a real physical button. The Task code runtime gets an event for the corresponding virtual device whenever the physical button is pressed.
In the “Denon driver” example above, an “on/off” virtual device can be bound to a real button on a remote.
Virtual or Not?
Although some virtual devices can be replaced with real devices in Thing-App design, some are indispensable.
If a device would not exist if the associated Thing-App task does not exist, then the device must be a virtual device.
If a device’s primary purpose is to get user input to the Thing-App, it should be a virtual device. For example, a Thing-App may need user input of an on/off signal that will affect its behavior; the device shall be a virtual device.
A virtual device can be further bound by an end-user with an unused physical real device, which makes virtual devices extremely flexible. So, the rule of thumb is that if a device can be a virtual device, it shall NOT be a real one.
Virtual Devie API
App Layer Transactions
Libertas follows the data model of Matter/Zigbee. The virtual device API is designed for developers to easily implement the application layer message transactions defined in the standard.
API List
Set device event callback function.
Device Transactions
Both devices and virtual devices can use the API calls below. But the behaviors are different.
For example, for a virtual device, Libertas_DeviceCommandReq
will send a command request to all bound devices of this virtual device. For a device, the function only sends the request to the corresponding device remotely.
The same logic applies to attribute transactions.